Main sections
08 Graphics (3D)
General
3D graphics is a complex issue. In GLBasic we tried to make the commands simple and easy to use like the 2D commands.
If you have not completed at least one 2D project, it is advisable that you do, 3D is a large step up from 2D. Jumping from compiling your first program directly into a 3D project could be an impossibly steep step for many new users.
GLBasic uses what is known as the right hand system - where Y always points upwards.
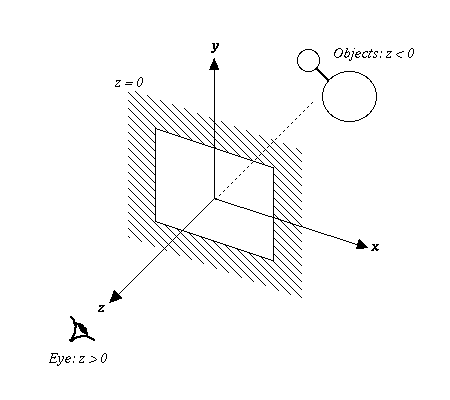
All 3D commands start with X_... so that you might find them very easily in your program and within this reference.
Please note that the 3D commands are only available as part GLBasic 3D upgrade.
Textures must be 2^n size (width or height must be 2, 4, 8, 16, 32, 65, 128, 256, 512...) Be aware that some models you may get from the internet will not feature this restriction. If this is the case with your models, then you will have to stretch the texture manually to an appropriate size - 64, 128, 256, 512 or 1024 pixels squared - otherwise ugly texture errors will occur.
Basically GLBasic 3D works like this:
<B>Switching to 3D mode:</B>
X_MAKE3D znear, zfar, field_of_view
Where znear and zfar describe the clipping range in a relative distance to the camera. Values of 1 and 1000 usually give good results. Bigger distances might cause a lack of quality when z-buffering the 3D scene. The field_of_view angle lets you specify the camera's zoom objective.
<B>Camera</B>
X_CAMERA x, y, z, px, py, pz
Sets the camera at position x, y, z and points it towards the point px, py, pz. Top is always along the positive Y axis.
<B>Light</B>
X_AMBIENT_LT num, col
Turns on an ambient light. 'Ambient' means light that shines from every direction. 'col' specifies the color of the light (See RGB).
'num' is the reference ID number of the light source. Values from 0-7 are allowed, since modern hardware doesn't support more than 8 lights at a time. If there are more than 9 light sources in a scene, the graphics card will try and use the 8 nearest lights. In professional applications no more than 2 lights are active within a scene at one time as it can cause the application to slow down.
X_SPOT_LT num, col, x, y, z, dirx, diry, dirz, cutoff
Creates a spot light at position x, y, z that shines in direction dirx, diry, dirz. The cutoff specifies the cut-off (width of the cone) angle of the spot light. The regular light in your scene will not (even if it seems a bit illogical in the beginning) cast any shadows. Instead the rendered triangle for the object will be drawn lighter or darker, depending on the angles between the camera, the triangle's normal, and the light's position.
<B>Transformations</B>
Now that the camera and lights are set, we need the most important thing, some 3D objects.
X_MOVEMENT x, y, z
X_SCALING fx, fy, fz
X_ROTATION phi, dx, dy, dz
X_MOVEMENT moves the current 3D drawing position to point x, y, z. The local origin of an object (0,0,0) will be moved to this position when drawing it.
X_SCALING scales an object factorizing the local axis. 'X_SCALING 0.5, 0.5, 0.5' for example halves the size of an object. 'X_SCALING 2, 2, 2' doubles the size of an object.
X_ROTATION rotates an object around the vector dx, dy, dz by angle phi. There can be several rotations queued up.
X_MOVEMENT or X_SCALING will reset all previously added rotations and must therefore be called before X_ROTATION.
<B>Objects</B>
The simplest way to create a 3D object within GLBasic is via loading a 3D file. You simply create it with a 3D program, export it in Quake '.md2' or 3D Studio '.3ds' format, and convert it to a compressed '.ddd' file with the 3DConvert tool. Once you have done this, you can load it as follows:
X_LOADOBJ filename$, idnum
Furthermore you can build objects at runtime with these commands.
X_OBJSTART idnum
X_OBJADDVERTEX x1, y1, z1, tx1, ty1, col
X_OBJADDVERTEX x2, y2, z2, tx2, ty2, col
X_OBJADDVERTEX x3, y3, z3, tx3, ty3, col
X_ENDOBJ
idnum specifies the reference ID number of the object. With this number you can draw it later. Existing object numbers can be overwritten.
X_OBJADDVERTEX adds a vertex to an object. tx, ty specify the texture coordinates (uv,uy - from the rang 0 to 1, 0.5 for instance is the center of the texture), '0'=top-left, '1'=bottom-right. The first 3 vectors form a triangle. Every additional vector forms a triangle with the previously last specified 2 vectors. If you don't want this method of drawing, you can 'lift the design pen' and restart with 3 independent vertices that for a new triangle by using the command.
X_OBJNEWGROUP
Now the loaded or created object must be drawn. Do this with:
X_DRAWOBJ idnum, frame
Whereby 'frame' is the index of the animation 'frame'. User defined objects have no animation, so you have to set it to '0' (i.e. the first frame)
You can play animations with :
X_DRAWANIM num, frame, from, upto, interpol, full_anim
'from' and 'upto' define the start and end frames for the animation. 'interpol' specifies how smooth the animation will be (interpolation step from 0 to 1). 'full_anim' indicates, that all frames in between 'from' and 'upto' will be used for animation. If you set it to FALSE, the frames will be interpolated directly. See the reference page for X_DRAWANIM for further details on the 'interpol' parameter.
In order to assign a texture to your object, you must have previously loaded a texture with LOADSPRITE or LOADBUMPTEXTURE and assign the texture with the X_SETTEXTURE command.
X_SETTEXTURE num, num_bump
'Num' represents the Sprite ID number, -1 indicates no texture should be used.
The parameter num_bump# specifies which normal map should be used for Dot3 Bump Mapping. The normal map can be created using LOADBUMPTEXTURE instead of LOADSPRITE. A value of -1 specifies that no bump mapping should be performed.
<B>Finishing 3D</B>
When working in 3D mode you cannot use any of the 2D commands. In order to use 2D commands you will have to change back to 2D mode with X_MAKE2D.
X_MAKE2D
<B>Special Rendering Modes</B>
If you want to use "Cel Shading", "Bump Mapping" or "Stencil Shadows", see the X_SPOT_LT function.
With X_SPHEREMAPPING you can program metallic reflections.